- Beranda
- Programmer Forum
Membuat class object sederhana c#
...
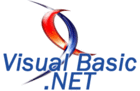
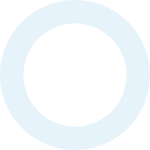
TS
free.lancer
Membuat class object sederhana c#
ane mau share cara membuat class c#
class di bawah ini bisa gunakan untuk membuat aplikasi desktop dan dan web..
//namespace
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
using Microsoft.ApplicationBlocks.Data;
public class Users
{
string _UserId, _FirstName, _LastName, _Password,_OldPassword, _CardId, _Status, _Email,_Comp, _Branch ,_CreatedBy,_UpdatedBy,_Remark;
DateTime _CreatedDate,_UpdatedDate;
private bool _Deleted, _Synch;
public string Sort_On;
clsDbConnector objdDbConnector = new clsDbConnector();
public Users()
{
//
// TODO: Add constructor logic here
//
}
public string UserID
{
get { return _UserId; }
set { _UserId = value; }
}
public string Password
{
get { return _Password; }
set { _Password = value; }
}
public string OldPassword
{
get { return _OldPassword; }
set { _OldPassword = value; }
}
public string FirstName
{
get { return _FirstName; }
set { _FirstName = value; }
}
public string LastName
{
get { return _LastName; }
set { _LastName = value; }
}
public string CardId
{
get { return _CardId; }
set { _CardId = value; }
}
public string Status
{
get { return _Status; }
set { _Status = value; }
}
public string Email
{
get { return _Email; }
set { _Email= value; }
}
public string Remark
{
get { return _Remark; }
set { _Remark = value; }
}
public string CreatedBy
{
get { return _CreatedBy; }
set { _CreatedBy = value; }
}
public DateTime CreatedDate
{
get { return _CreatedDate; }
set { _CreatedDate = value; }
}
public string UpdatedBy
{
get { return _UpdatedBy; }
set { _UpdatedBy = value; }
}
public DateTime UpdatedDate
{
get { return _UpdatedDate; }
set { _UpdatedDate = value; }
}
public string Comp
{
get { return _Comp; }
set { _Comp = value; }
}
public string Branch
{
get { return _Branch; }
set { _Branch = value; }
}
public bool Deleted
{
get { return _Deleted; }
set { _Deleted = value; }
}
public bool Synch
{
get { return _Synch; }
set { _Synch = value; }
}
public DataSet GetUserLoginDetails()
{
SqlParameter[] p = new SqlParameter[4];
p[0] = new SqlParameter("@userId", SqlDbType.VarChar);
p[0].Value = this._UserId;
p[1] = new SqlParameter("@password", SqlDbType.VarChar);
p[1].Value = this._Password;
p[2] = new SqlParameter("@comp", SqlDbType.VarChar);
p[2].Value = this._Comp;
p[3] = new SqlParameter("@branch", SqlDbType.VarChar);
p[3].Value = this._Branch;
DataSet dsUserLoginDetails = SqlHelper.ExecuteDataset(ClsConnectionString.getConnectionString(), CommandType.StoredProcedure, "spUserLogin", p);
return dsUserLoginDetails;
}
public void GetUserDetails()
{
string strSql = string.Empty;
strSql += "select * from [user] where ";
strSql += " UserId='" + _UserId + "'";
strSql += " and companyId='" + _Comp + "'";
strSql += " and branchId='" + _Branch + "'";
DataSet ds=SqlHelper.ExecuteDataset(ClsConnectionString.getConnectionString(),CommandType.Text,strSql);
DataRowCollection drc = ds.Tables[0].Rows;
if (drc.Count > 0)
{
_UserId = drc[0]["userId"].ToString();
_FirstName = drc[0]["firstName"].ToString();
_LastName= drc[0]["lastName"].ToString();
_Password = drc[0]["password"].ToString();
_OldPassword = drc[0]["password"].ToString();
_Status = drc[0]["status"].ToString();
_CardId = drc[0]["cardId"].ToString();
_Email = drc[0]["email"].ToString();
_CreatedBy = drc[0]["createdBy"].ToString();
_CreatedDate = Convert.ToDateTime(drc[0]["createdDate"].ToString());
_UpdatedBy = drc[0]["updatedBy"].ToString();
_UpdatedDate = Convert.ToDateTime(drc[0]["updatedDate"].ToString());
_Comp = drc[0]["companyId"].ToString();
_Branch = drc[0]["BranchId"].ToString();
_Deleted = Convert.ToBoolean(drc[0]["deleted"].ToString());
_Synch = Convert.ToBoolean(drc[0]["synch"].ToString());
_Remark = drc[0]["remark"].ToString();
}
else
{
_UserId = "";
_FirstName ="";
_LastName = "";
_Password = "";
_OldPassword = "";
_Status = "";
_CardId = "";
_Email = "";
_CreatedBy = "";
_CreatedDate = DateTime.Now;
_UpdatedBy = "";
_UpdatedDate = DateTime.Now;
_Comp = "";
_Branch = "";
_Deleted = false;
_Synch = false;
_Remark = "";
}
}
public DataSet GetUser()
{
string strSql = string.Empty;
strSql = "select *,(firstname + ' ' + lastName) as fullName from [user] where companyId='"+ _Comp +"' and branchId='"+_Branch +"'";
if (!string.IsNullOrEmpty(_UserId))
strSql += " and userId like '%" + _UserId + "%'";
if (!string.IsNullOrEmpty(_FirstName))
strSql += " and firstName like '%" + _FirstName + "%'";
if (!string.IsNullOrEmpty(_LastName))
strSql += " and lastName like '%" + _LastName + "%'";
if (!string.IsNullOrEmpty(_Status))
strSql += " and status like '%" + _Status + "%'";
if (!string.IsNullOrEmpty(Sort_On))
{
strSql = strSql + " ORDER BY " + Sort_On;
}
else
{
strSql = strSql + " Order By firstName ASC ";
}
DataSet dsTemp;
dsTemp = SqlHelper.ExecuteDataset(ClsConnectionString.getConnectionString(), CommandType.Text, strSql);
return dsTemp;
}
public void DeleteUser(string UserId,string comp, string branch)
{
String[] uid = UserId.Split(',');
if (uid.Length > 0)
{
for (int i = 0; i < uid.Length; i++){
string strSql;
strSql = string.Empty;
strSql += "delete from userAccessControl where UserId ='" + uid[i].ToString() + "'";
strSql += " and companyId='" + comp + "'";
strSql += " and branchId='" + branch + "'";
strSql += ";delete from [user] where UserId ='" + uid[i].ToString() + "'";
strSql += " and companyId='" + comp + "'";
strSql += " and branchId='" + branch + "'";
SqlHelper.ExecuteNonQuery(ClsConnectionString.getConnectionString(), CommandType.Text, strSql);
}
}
else {
string strSql;
strSql = string.Empty;
strSql += "delete from userAccessControl where UserId ='" + UserId + "'";
strSql += " and companyId='" + comp + "'";
strSql += " and branchId='" + branch + "'";
strSql += ";delete from [user] where UserId ='" + UserId + "'";
strSql += " and companyId='" + comp + "'";
strSql += " and branchId='" + branch + "'";
SqlHelper.ExecuteNonQuery(ClsConnectionString.getConnectionString(), CommandType.Text, strSql);
}
}
public void AddUser()
{
SqlParameter[] p = new SqlParameter[16];
p[0] = new SqlParameter("@userId", SqlDbType.VarChar);
p[0].Value=_UserId;
p[1] = new SqlParameter("@firstName", SqlDbType.VarChar);
p[1].Value = _FirstName;
p[2] = new SqlParameter("@lastName", SqlDbType.VarChar);
p[2].Value = _LastName;
p[3] = new SqlParameter("@password", SqlDbType.VarChar);
p[3].Value = _Password;
p[4] = new SqlParameter("@cardId", SqlDbType.VarChar);
p[4].Value = _CardId;
p[5] = new SqlParameter("@status", SqlDbType.VarChar);
p[5].Value = _Status;
p[6] = new SqlParameter("@createdBy", SqlDbType.VarChar);
p[6].Value = _CreatedBy;
p[7] = new SqlParameter("@createdDate", SqlDbType.DateTime);
p[7].Value = _CreatedDate;
p[8] = new SqlParameter("@updatedBy", SqlDbType.VarChar);
p[8].Value = _UpdatedBy;
p[9] = new SqlParameter("@updatedDate", SqlDbType.DateTime);
p[9].Value = _UpdatedDate;
p[10] = new SqlParameter("@email", SqlDbType.VarChar);
p[10].Value = _Email;
p[11] = new SqlParameter("@remark", SqlDbType.VarChar);
p[11].Value = _Remark;
p[12] = new SqlParameter("@companyId", SqlDbType.VarChar);
p[12].Value = _Comp;
p[13] = new SqlParameter("@branchId", SqlDbType.VarChar);
p[13].Value = _Branch;
p[14] = new SqlParameter("@deleted", SqlDbType.Bit);
p[14].Value = _Deleted;
p[15] = new SqlParameter("@synch", SqlDbType.Bit);
p[15].Value = _Synch;
SqlHelper.ExecuteNonQuery(ClsConnectionString.getConnectionString(), CommandType.StoredProcedure, "spAddUser", p);
}
public void UpdateUser()
{
SqlParameter[] p = new SqlParameter[12];
p[0] = new SqlParameter("@userId", SqlDbType.VarChar);
p[0].Value = _UserId;
p[1] = new SqlParameter("@firstName", SqlDbType.VarChar);
p[1].Value = _FirstName;
p[2] = new SqlParameter("@lastName", SqlDbType.VarChar);
p[2].Value = _LastName;
p[3] = new SqlParameter("@password", SqlDbType.VarChar);
p[3].Value = _Password;
p[4] = new SqlParameter("@cardId", SqlDbType.VarChar);
p[4].Value = _CardId;
p[5] = new SqlParameter("@status", SqlDbType.VarChar);
p[5].Value = _Status;
p[6] = new SqlParameter("@updatedBy", SqlDbType.VarChar);
p[6].Value = _UpdatedBy;
p[7] = new SqlParameter("@updatedDate", SqlDbType.DateTime);
p[7].Value = _UpdatedDate;
p[8] = new SqlParameter("@email", SqlDbType.VarChar);
p[8].Value = _Email;
p[9] = new SqlParameter("@remark", SqlDbType.VarChar);
p[9].Value = _Remark;
p[10] = new SqlParameter("@companyId", SqlDbType.VarChar);
p[10].Value = _Comp;
p[11] = new SqlParameter("@branchId", SqlDbType.VarChar);
p[11].Value = _Branch;
SqlHelper.ExecuteNonQuery(ClsConnectionString.getConnectionString(), CommandType.StoredProcedure, "spUpdateUser", p);
}
public bool CheckUserName()
{
SqlParameter[] p = new SqlParameter[1];
p[0] = new SqlParameter("@userId", SqlDbType.VarChar);
p[0].Value = _UserId;
DataSet ds = SqlHelper.ExecuteDataset(ClsConnectionString.getConnectionString(), CommandType.StoredProcedure, "spCheckUserName", p);
DataTable dtable = ds.Tables[0];
if (dtable.Rows.Count > 0)
return true;
else
return false;
}
public int UserChangePassword()
{
SqlParameter[] p = new SqlParameter[2];
p[0] = new SqlParameter("@UserId", SqlDbType.VarChar);
p[0].Value = this._UserId;
p[1] = new SqlParameter("@Password", SqlDbType.VarChar);
p[1].Value = this._Password;
int i = SqlHelper.ExecuteNonQuery(ClsConnectionString.getConnectionString(), CommandType.StoredProcedure, "spUserChangePassword", p);
return i;
}
public bool UserCheckPassword()
{
SqlParameter[] p = new SqlParameter[2];
p[0] = new SqlParameter("@UserId", SqlDbType.VarChar);
p[0].Value = this._UserId;
p[1] = new SqlParameter("@Password", SqlDbType.VarChar);
p[1].Value = _OldPassword;
DataSet ds = SqlHelper.ExecuteDataset(ClsConnectionString.getConnectionString(), CommandType.StoredProcedure, "spUserCheckPassword", p);
DataRowCollection drc = ds.Tables[0].Rows;
if (drc.Count > 0)
{
return true;
}
else
return false;
}
public bool checkUserIdForGetPwd()
{
string STRSQL = " SELECT userId,password,email FROM user ";
STRSQL = STRSQL + " WHERE userId='" + _UserId + "'";
STRSQL = STRSQL + " and companyId='" + _Comp + "'";
STRSQL = STRSQL + " and branchId='" + _Branch + "'";
DataSet dsTemp;
dsTemp = objdDbConnector.GetDataSet(STRSQL);
DataRowCollection dRC = dsTemp.Tables[0].Rows;
if (dRC.Count > 0)
{
DataRow dR = dRC[0];
_UserId = dR["userId"].ToString();
_Password = dR["Password"].ToString();
if (!String.IsNullOrEmpty(dR["Email"].ToString()))
_Email = dR["Email"].ToString();
return true;
}
return false;
}
}
class di bawah ini bisa gunakan untuk membuat aplikasi desktop dan dan web..
//namespace
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
using Microsoft.ApplicationBlocks.Data;
public class Users
{
string _UserId, _FirstName, _LastName, _Password,_OldPassword, _CardId, _Status, _Email,_Comp, _Branch ,_CreatedBy,_UpdatedBy,_Remark;
DateTime _CreatedDate,_UpdatedDate;
private bool _Deleted, _Synch;
public string Sort_On;
clsDbConnector objdDbConnector = new clsDbConnector();
public Users()
{
//
// TODO: Add constructor logic here
//
}
public string UserID
{
get { return _UserId; }
set { _UserId = value; }
}
public string Password
{
get { return _Password; }
set { _Password = value; }
}
public string OldPassword
{
get { return _OldPassword; }
set { _OldPassword = value; }
}
public string FirstName
{
get { return _FirstName; }
set { _FirstName = value; }
}
public string LastName
{
get { return _LastName; }
set { _LastName = value; }
}
public string CardId
{
get { return _CardId; }
set { _CardId = value; }
}
public string Status
{
get { return _Status; }
set { _Status = value; }
}
public string Email
{
get { return _Email; }
set { _Email= value; }
}
public string Remark
{
get { return _Remark; }
set { _Remark = value; }
}
public string CreatedBy
{
get { return _CreatedBy; }
set { _CreatedBy = value; }
}
public DateTime CreatedDate
{
get { return _CreatedDate; }
set { _CreatedDate = value; }
}
public string UpdatedBy
{
get { return _UpdatedBy; }
set { _UpdatedBy = value; }
}
public DateTime UpdatedDate
{
get { return _UpdatedDate; }
set { _UpdatedDate = value; }
}
public string Comp
{
get { return _Comp; }
set { _Comp = value; }
}
public string Branch
{
get { return _Branch; }
set { _Branch = value; }
}
public bool Deleted
{
get { return _Deleted; }
set { _Deleted = value; }
}
public bool Synch
{
get { return _Synch; }
set { _Synch = value; }
}
public DataSet GetUserLoginDetails()
{
SqlParameter[] p = new SqlParameter[4];
p[0] = new SqlParameter("@userId", SqlDbType.VarChar);
p[0].Value = this._UserId;
p[1] = new SqlParameter("@password", SqlDbType.VarChar);
p[1].Value = this._Password;
p[2] = new SqlParameter("@comp", SqlDbType.VarChar);
p[2].Value = this._Comp;
p[3] = new SqlParameter("@branch", SqlDbType.VarChar);
p[3].Value = this._Branch;
DataSet dsUserLoginDetails = SqlHelper.ExecuteDataset(ClsConnectionString.getConnectionString(), CommandType.StoredProcedure, "spUserLogin", p);
return dsUserLoginDetails;
}
public void GetUserDetails()
{
string strSql = string.Empty;
strSql += "select * from [user] where ";
strSql += " UserId='" + _UserId + "'";
strSql += " and companyId='" + _Comp + "'";
strSql += " and branchId='" + _Branch + "'";
DataSet ds=SqlHelper.ExecuteDataset(ClsConnectionString.getConnectionString(),CommandType.Text,strSql);
DataRowCollection drc = ds.Tables[0].Rows;
if (drc.Count > 0)
{
_UserId = drc[0]["userId"].ToString();
_FirstName = drc[0]["firstName"].ToString();
_LastName= drc[0]["lastName"].ToString();
_Password = drc[0]["password"].ToString();
_OldPassword = drc[0]["password"].ToString();
_Status = drc[0]["status"].ToString();
_CardId = drc[0]["cardId"].ToString();
_Email = drc[0]["email"].ToString();
_CreatedBy = drc[0]["createdBy"].ToString();
_CreatedDate = Convert.ToDateTime(drc[0]["createdDate"].ToString());
_UpdatedBy = drc[0]["updatedBy"].ToString();
_UpdatedDate = Convert.ToDateTime(drc[0]["updatedDate"].ToString());
_Comp = drc[0]["companyId"].ToString();
_Branch = drc[0]["BranchId"].ToString();
_Deleted = Convert.ToBoolean(drc[0]["deleted"].ToString());
_Synch = Convert.ToBoolean(drc[0]["synch"].ToString());
_Remark = drc[0]["remark"].ToString();
}
else
{
_UserId = "";
_FirstName ="";
_LastName = "";
_Password = "";
_OldPassword = "";
_Status = "";
_CardId = "";
_Email = "";
_CreatedBy = "";
_CreatedDate = DateTime.Now;
_UpdatedBy = "";
_UpdatedDate = DateTime.Now;
_Comp = "";
_Branch = "";
_Deleted = false;
_Synch = false;
_Remark = "";
}
}
public DataSet GetUser()
{
string strSql = string.Empty;
strSql = "select *,(firstname + ' ' + lastName) as fullName from [user] where companyId='"+ _Comp +"' and branchId='"+_Branch +"'";
if (!string.IsNullOrEmpty(_UserId))
strSql += " and userId like '%" + _UserId + "%'";
if (!string.IsNullOrEmpty(_FirstName))
strSql += " and firstName like '%" + _FirstName + "%'";
if (!string.IsNullOrEmpty(_LastName))
strSql += " and lastName like '%" + _LastName + "%'";
if (!string.IsNullOrEmpty(_Status))
strSql += " and status like '%" + _Status + "%'";
if (!string.IsNullOrEmpty(Sort_On))
{
strSql = strSql + " ORDER BY " + Sort_On;
}
else
{
strSql = strSql + " Order By firstName ASC ";
}
DataSet dsTemp;
dsTemp = SqlHelper.ExecuteDataset(ClsConnectionString.getConnectionString(), CommandType.Text, strSql);
return dsTemp;
}
public void DeleteUser(string UserId,string comp, string branch)
{
String[] uid = UserId.Split(',');
if (uid.Length > 0)
{
for (int i = 0; i < uid.Length; i++){
string strSql;
strSql = string.Empty;
strSql += "delete from userAccessControl where UserId ='" + uid[i].ToString() + "'";
strSql += " and companyId='" + comp + "'";
strSql += " and branchId='" + branch + "'";
strSql += ";delete from [user] where UserId ='" + uid[i].ToString() + "'";
strSql += " and companyId='" + comp + "'";
strSql += " and branchId='" + branch + "'";
SqlHelper.ExecuteNonQuery(ClsConnectionString.getConnectionString(), CommandType.Text, strSql);
}
}
else {
string strSql;
strSql = string.Empty;
strSql += "delete from userAccessControl where UserId ='" + UserId + "'";
strSql += " and companyId='" + comp + "'";
strSql += " and branchId='" + branch + "'";
strSql += ";delete from [user] where UserId ='" + UserId + "'";
strSql += " and companyId='" + comp + "'";
strSql += " and branchId='" + branch + "'";
SqlHelper.ExecuteNonQuery(ClsConnectionString.getConnectionString(), CommandType.Text, strSql);
}
}
public void AddUser()
{
SqlParameter[] p = new SqlParameter[16];
p[0] = new SqlParameter("@userId", SqlDbType.VarChar);
p[0].Value=_UserId;
p[1] = new SqlParameter("@firstName", SqlDbType.VarChar);
p[1].Value = _FirstName;
p[2] = new SqlParameter("@lastName", SqlDbType.VarChar);
p[2].Value = _LastName;
p[3] = new SqlParameter("@password", SqlDbType.VarChar);
p[3].Value = _Password;
p[4] = new SqlParameter("@cardId", SqlDbType.VarChar);
p[4].Value = _CardId;
p[5] = new SqlParameter("@status", SqlDbType.VarChar);
p[5].Value = _Status;
p[6] = new SqlParameter("@createdBy", SqlDbType.VarChar);
p[6].Value = _CreatedBy;
p[7] = new SqlParameter("@createdDate", SqlDbType.DateTime);
p[7].Value = _CreatedDate;
p[8] = new SqlParameter("@updatedBy", SqlDbType.VarChar);
p[8].Value = _UpdatedBy;
p[9] = new SqlParameter("@updatedDate", SqlDbType.DateTime);
p[9].Value = _UpdatedDate;
p[10] = new SqlParameter("@email", SqlDbType.VarChar);
p[10].Value = _Email;
p[11] = new SqlParameter("@remark", SqlDbType.VarChar);
p[11].Value = _Remark;
p[12] = new SqlParameter("@companyId", SqlDbType.VarChar);
p[12].Value = _Comp;
p[13] = new SqlParameter("@branchId", SqlDbType.VarChar);
p[13].Value = _Branch;
p[14] = new SqlParameter("@deleted", SqlDbType.Bit);
p[14].Value = _Deleted;
p[15] = new SqlParameter("@synch", SqlDbType.Bit);
p[15].Value = _Synch;
SqlHelper.ExecuteNonQuery(ClsConnectionString.getConnectionString(), CommandType.StoredProcedure, "spAddUser", p);
}
public void UpdateUser()
{
SqlParameter[] p = new SqlParameter[12];
p[0] = new SqlParameter("@userId", SqlDbType.VarChar);
p[0].Value = _UserId;
p[1] = new SqlParameter("@firstName", SqlDbType.VarChar);
p[1].Value = _FirstName;
p[2] = new SqlParameter("@lastName", SqlDbType.VarChar);
p[2].Value = _LastName;
p[3] = new SqlParameter("@password", SqlDbType.VarChar);
p[3].Value = _Password;
p[4] = new SqlParameter("@cardId", SqlDbType.VarChar);
p[4].Value = _CardId;
p[5] = new SqlParameter("@status", SqlDbType.VarChar);
p[5].Value = _Status;
p[6] = new SqlParameter("@updatedBy", SqlDbType.VarChar);
p[6].Value = _UpdatedBy;
p[7] = new SqlParameter("@updatedDate", SqlDbType.DateTime);
p[7].Value = _UpdatedDate;
p[8] = new SqlParameter("@email", SqlDbType.VarChar);
p[8].Value = _Email;
p[9] = new SqlParameter("@remark", SqlDbType.VarChar);
p[9].Value = _Remark;
p[10] = new SqlParameter("@companyId", SqlDbType.VarChar);
p[10].Value = _Comp;
p[11] = new SqlParameter("@branchId", SqlDbType.VarChar);
p[11].Value = _Branch;
SqlHelper.ExecuteNonQuery(ClsConnectionString.getConnectionString(), CommandType.StoredProcedure, "spUpdateUser", p);
}
public bool CheckUserName()
{
SqlParameter[] p = new SqlParameter[1];
p[0] = new SqlParameter("@userId", SqlDbType.VarChar);
p[0].Value = _UserId;
DataSet ds = SqlHelper.ExecuteDataset(ClsConnectionString.getConnectionString(), CommandType.StoredProcedure, "spCheckUserName", p);
DataTable dtable = ds.Tables[0];
if (dtable.Rows.Count > 0)
return true;
else
return false;
}
public int UserChangePassword()
{
SqlParameter[] p = new SqlParameter[2];
p[0] = new SqlParameter("@UserId", SqlDbType.VarChar);
p[0].Value = this._UserId;
p[1] = new SqlParameter("@Password", SqlDbType.VarChar);
p[1].Value = this._Password;
int i = SqlHelper.ExecuteNonQuery(ClsConnectionString.getConnectionString(), CommandType.StoredProcedure, "spUserChangePassword", p);
return i;
}
public bool UserCheckPassword()
{
SqlParameter[] p = new SqlParameter[2];
p[0] = new SqlParameter("@UserId", SqlDbType.VarChar);
p[0].Value = this._UserId;
p[1] = new SqlParameter("@Password", SqlDbType.VarChar);
p[1].Value = _OldPassword;
DataSet ds = SqlHelper.ExecuteDataset(ClsConnectionString.getConnectionString(), CommandType.StoredProcedure, "spUserCheckPassword", p);
DataRowCollection drc = ds.Tables[0].Rows;
if (drc.Count > 0)
{
return true;
}
else
return false;
}
public bool checkUserIdForGetPwd()
{
string STRSQL = " SELECT userId,password,email FROM user ";
STRSQL = STRSQL + " WHERE userId='" + _UserId + "'";
STRSQL = STRSQL + " and companyId='" + _Comp + "'";
STRSQL = STRSQL + " and branchId='" + _Branch + "'";
DataSet dsTemp;
dsTemp = objdDbConnector.GetDataSet(STRSQL);
DataRowCollection dRC = dsTemp.Tables[0].Rows;
if (dRC.Count > 0)
{
DataRow dR = dRC[0];
_UserId = dR["userId"].ToString();
_Password = dR["Password"].ToString();
if (!String.IsNullOrEmpty(dR["Email"].ToString()))
_Email = dR["Email"].ToString();
return true;
}
return false;
}
}
0
766
0
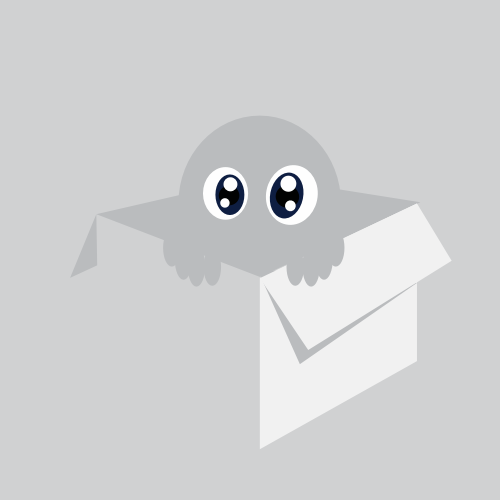
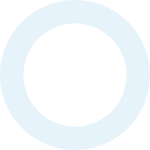
Guest
Tulis komentar menarik atau mention replykgpt untuk ngobrol seru
Mari bergabung, dapatkan informasi dan teman baru!

Programmer Forum
20.2KThread•4.3KAnggota
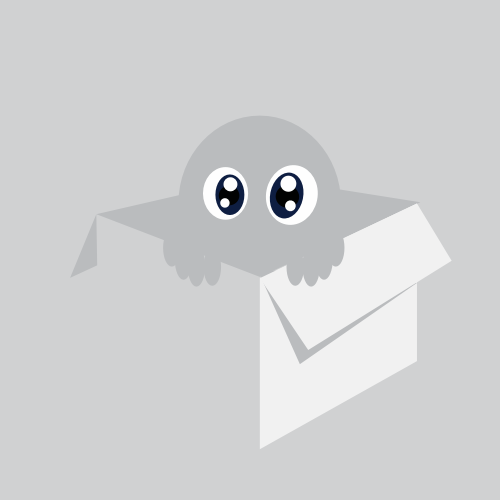
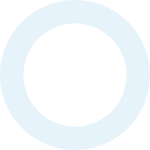
Guest
Tulis komentar menarik atau mention replykgpt untuk ngobrol seru